import {
ConversationEmbed,
AuthType,
init,
prefetch,
}
from '@thoughtspot/visual-embed-sdk';
Embed Spotter Beta
ThoughtSpot supports Natural Language Search queries and AI-generated Answers. With ThoughtSpot Spotter, this experience is further enhanced to a full conversational interface that users can interact with, ask follow-up questions, and get insights.
Embedding applications can avail the benefits of Spotter by using the following features:
-
Embed Spotter capabilities using Visual Embed SDK
-
Use REST APIs to create a conversation session, ask follow-up questions, and generate Answers
-
Integrate Spotter capabilities into your chatbot
Note
|
The Spotter feature is in beta and disabled by default on ThoughtSpot Embedded instances. To enable this feature on your instance, contact ThoughtSpot Support. |
Embed Spotter using Visual Embed SDKπ
Visual Embed SDK offers several options to seamlessly embed conversational analytics within your applications and customize the interface and experience as per your organizationβs branding guidelines.
If you want to embed only the conversation interface, you can use the ConversationEmbed
package and add it to your application.
Note
|
If you are embedding full ThoughtSpot experience in your app using |
Spotter componentsπ
The Spotter embedding adds the following components to your embedding application:
-
Datasource selector that allows users to select a data source for conversation with Spotter.
-
Preview data link that allows users to preview the contents of the data source used for the conversation.
-
Input box to ask a question to Spotter
-
Reset button to reset the chat conversation
-
Charts and tables generated by Spotter in response to a query
-
Menu actions such as Pin, Save, and Download, and a toggle switch to switch between chart and tabular format
Developers can use the customization features in the SDK to customize the look and feel of the interface, and include or exclude menu elements on the conversation interface.
Get started with embedding Spotterπ
Before you begin, check the following:
-
Spotter is enabled on your ThoughtSpot instance
-
Your environment has Visual Embed SDK v1.33.1 or later version
Import the SDK packageπ
Import the ConversationEmbed
SDK library to your application environment:
npm
ES6
<script type = 'module'>
import {
ConversationEmbed,
AuthType,
init,
prefetch,
}
from 'https://cdn.jsdelivr.net/npm/@thoughtspot/visual-embed-sdk/dist/index.js';
Initialize the SDKπ
To initialize the SDK, the following information is required:
-
thoughtSpotHost
The hostname of your ThoughtSpot application instance. See FAQs to know how to find the hostname of your application instance. -
authType
Authentication type. ThoughtSpot supports a variety of Authentication types. For testing purposes, you can useAuthType.None
. For other authentication options, see Authentication.
init({
thoughtSpotHost: '<%=tshost%>',
authType: AuthType.None,
});
Create an instance of ConversationEmbed objectπ
Create an instance of the ConversationEmbed
object and specify the data source ID. Optionally, you can specify the search query string to generate a chart or table at load time.
const conversation = new ConversationEmbed(document.getElementById('ts-embed'), {
frameParams: {
width: '100%',
height: '100%',
},
worksheetId: '<%=datasourceGUID%>',
searchOptions: {
searchQuery: 'sales by region',
},
});
const conversation = new ConversationEmbed(document.getElementById('ts-embed'), {
frameParams: {
width: '100%',
height: '100%',
},
worksheetId: '<%=datasourceGUID%>',
searchOptions: {
searchQuery: 'sales by region',
},
});
Customize your embed (Optional)π
To customize the Spotter interface, use the configuration attributes and properties available for ConversationEmbed
embed in the SDK.
Customize style, icons, and textπ
To customize the look and feel of the Spotter page, you can use the customizations
settings in the SDK. The customizations
object allows you to add custom CSS definitions, text strings, and icons.
For example, if you want to use your own icon sprites and the SVG for the icons is hosted on a Web server, you can replace the default icons on the spotter page as shown in this example.
init({
//...
customizations: {
iconSpriteUrl: "https://cdn.jsdelivr.net/gh/thoughtspot/custom-css-demo/alternate-spotter-icon.svg"
}
});
Note
|
When customizing icons, ensure that the hosting server is added to to the CSP connect-src domains list on the Develop > Security Settings page. For more information, see Security Settings. |
Customize menu elementsπ
You can show or hide menu elements such as the Pin, Save, and Download buttons, and chart switcher icon on the Spotter page using disabledActions
, visibleActions
, or hiddenActions
array as shown in this example:
//...
disabledActions: [Action.Pin,Action.Save]
Note
|
Action enumerators to show, disable, or hide menu elements such as Preview data, Reset, feedback widget, and the edit and delete icons on the Spotter page are not available in Visual Embed SDK for ThoughtSpot 10.5.0.cl release. |
Register, handle, and trigger eventsπ
Register event listeners.
conversation.on(EmbedEvent.Init, showLoader)
conversation.on(EmbedEvent.Load, hideLoader)
For more information about event types, see the following pages:
Render the embedded objectπ
conversation.render();
Test your embeddingπ
Load the embedded object in your app. If the embedding is successful, you will see the following page:
Spotter pageπ
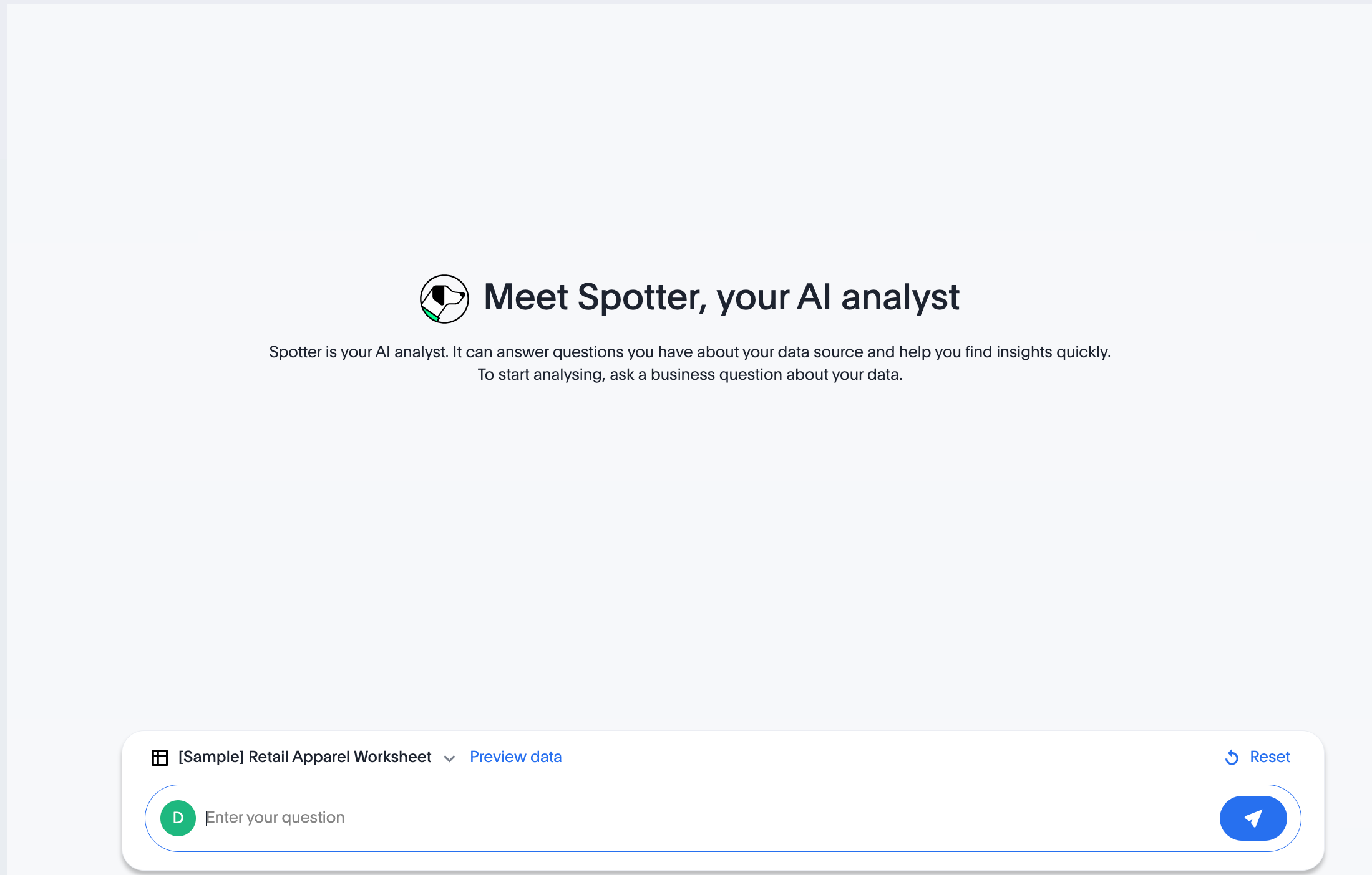
Spotter page with the preloaded query and search resultπ
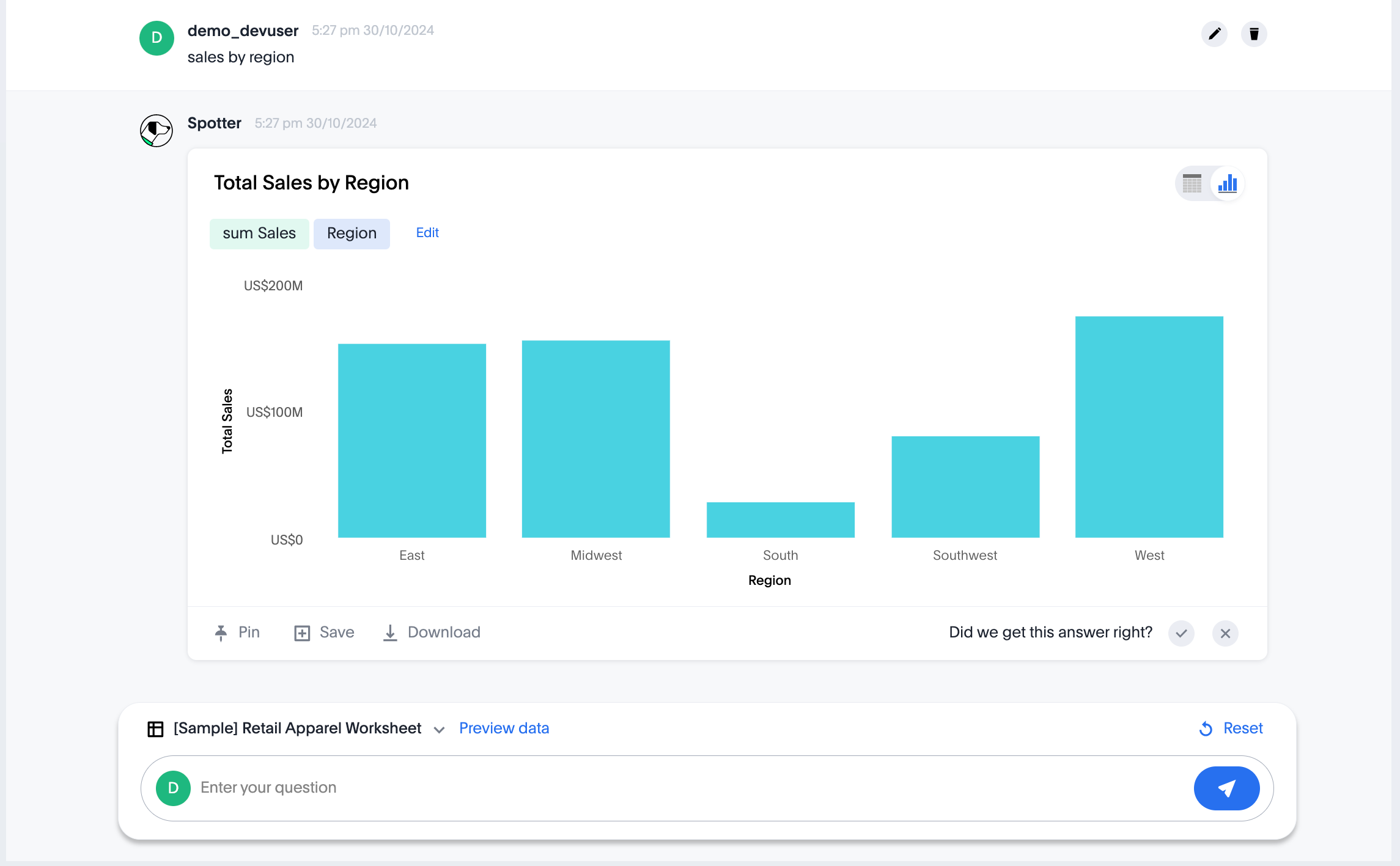
Spotter page with custom icon and textπ
The following figures show the Spotter page with custom icon sprite and text:
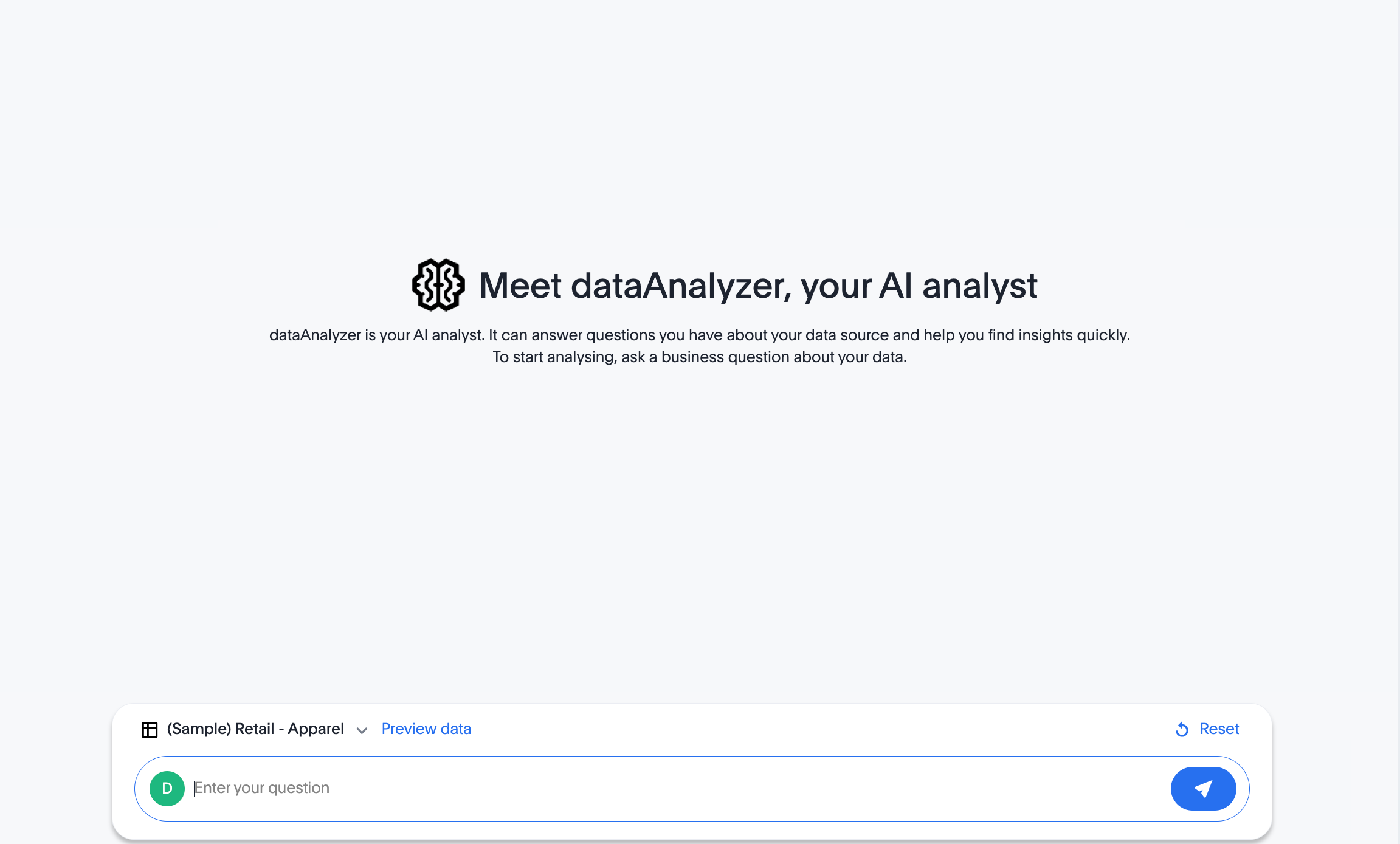
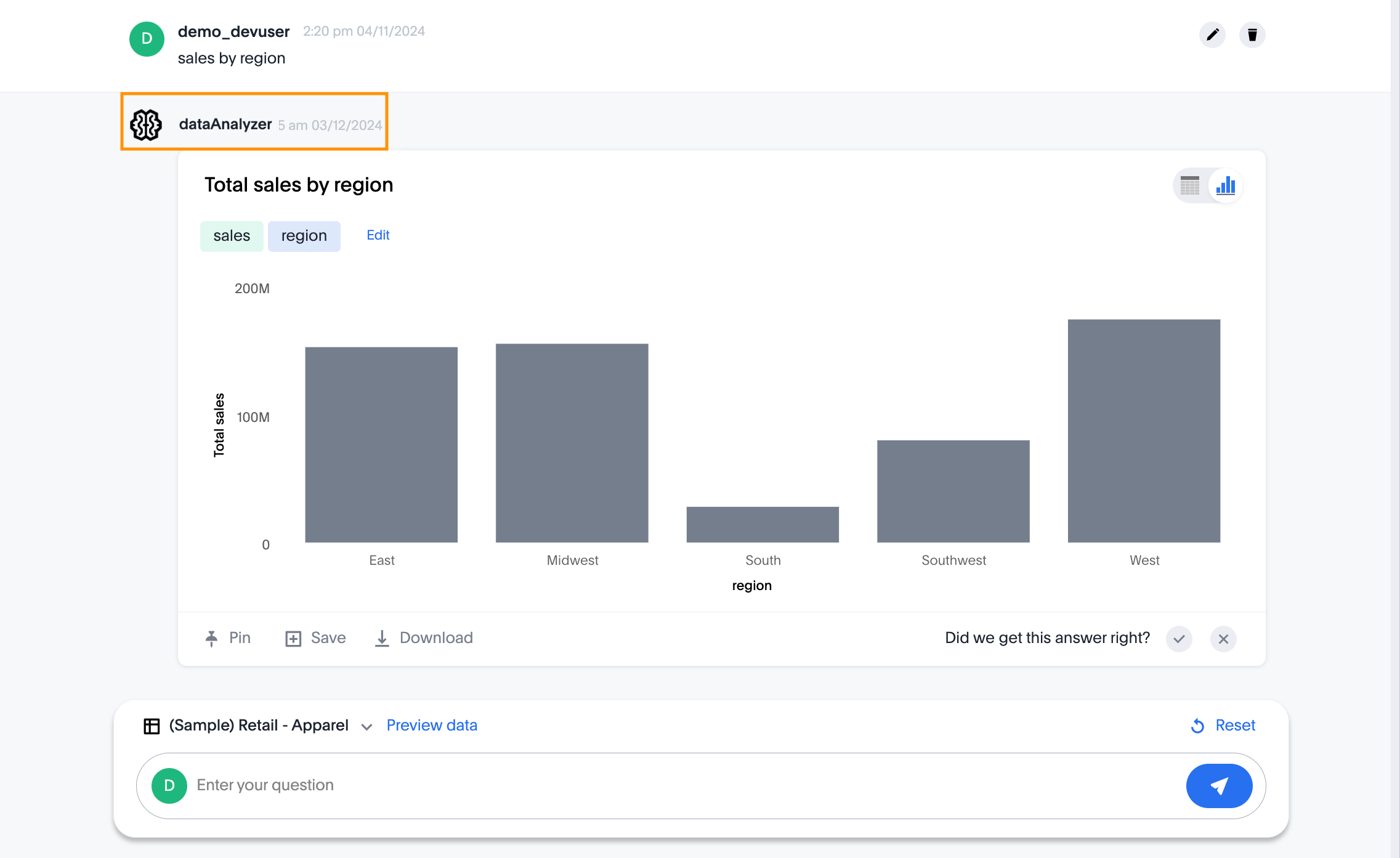