import {
LiveboardEmbed,
AuthType,
init,
prefetch,
EmbedEvent
}
from '@thoughtspot/visual-embed-sdk';
Embed a Liveboard
- Import the LiveboardEmbed package
- Initialize the SDK
- Create an instance of the LiveboardEmbed class
- Customize component via LiveboardViewConfig
- Register, handle, and trigger events
- Render the embedded Liveboard
- Verify the embedded object
- Common customization options
- Liveboard experience
- Additional resources
This page explains how to embed a ThoughtSpot Liveboard in your Web page, portal, or application.
A ThoughtSpot Liveboard is an interactive dashboard that presents a collection of visualizations pinned by a user.
Import the LiveboardEmbed package🔗
Import the LiveboardEmbed
SDK library to your application environment:
npm
ES6
<script type = 'module'>
import {
LiveboardEmbed,
AuthType,
init,
prefetch
}
from 'https://cdn.jsdelivr.net/npm/@thoughtspot/visual-embed-sdk/dist/tsembed.es.js';
Initialize the SDK🔗
Initialize the SDK and define authentication attributes.
Create an instance of the LiveboardEmbed class🔗
const liveboardEmbed = new LiveboardEmbed(document.getElementById('ts-embed'), {
frameParams: {
width: '100%',
height: '100%',
},
liveboardId: '<%=liveboardGUID%>',
});
For more information about the Liveboard embed object, classes, methods, interface properties, and enumeration members, see the following pages:
Customize component via LiveboardViewConfig🔗
The second argument of the LiveboardEmbed()
constructor is a LiveboardViewConfig
object. There are a number of properties that affect the display of the UI around the Liveboard and even the display of the Liveboard itself.
The disabledActions
, hiddenActions
, and visibleActions
properties of the LiveboardViewConfig
object all take an array of Actions items to control which menu items and buttons display.
For information about the frequently used properties, see common customizations.
Register, handle, and trigger events🔗
Register event listeners.
liveboardEmbed.on(EmbedEvent.init, showLoader)
liveboardEmbed.on(EmbedEvent.load, hideLoader)
For more information about events, see the following pages:
Render the embedded Liveboard🔗
Render the embedded Liveboard.
liveboardEmbed.render();
Verify the embedded object🔗
-
Load the embedded object in your app. If the embedding is successful, you will see a Liveboard page with visualizations.
-
Explore the charts and tables, and verify if objects render and show the desired data.
Common customization options🔗
Within the LiveboardViewConfig, there are several categories of properties that are frequently used to customize the LiveboardEmbed
component to fit within the embedding app’s UI experience.
Show/hide large UI elements🔗
Parameters such as hideLiveboardHeader
, hideTabPanel
, isLiveboardHeaderSticky
, showLiveboardTitle
, and showLiveboardDescription
control various aspects of the standard embedded Liveboard experience. Note the phrasing of the property name and the description in the documentation to understand whether true
enables or disables the particular feature.
Along with Actions there is very fine-grained control of the display of the LiveboardEmbed
component, which can be varied for each user or content object displayed depending on the desires of the app development team.
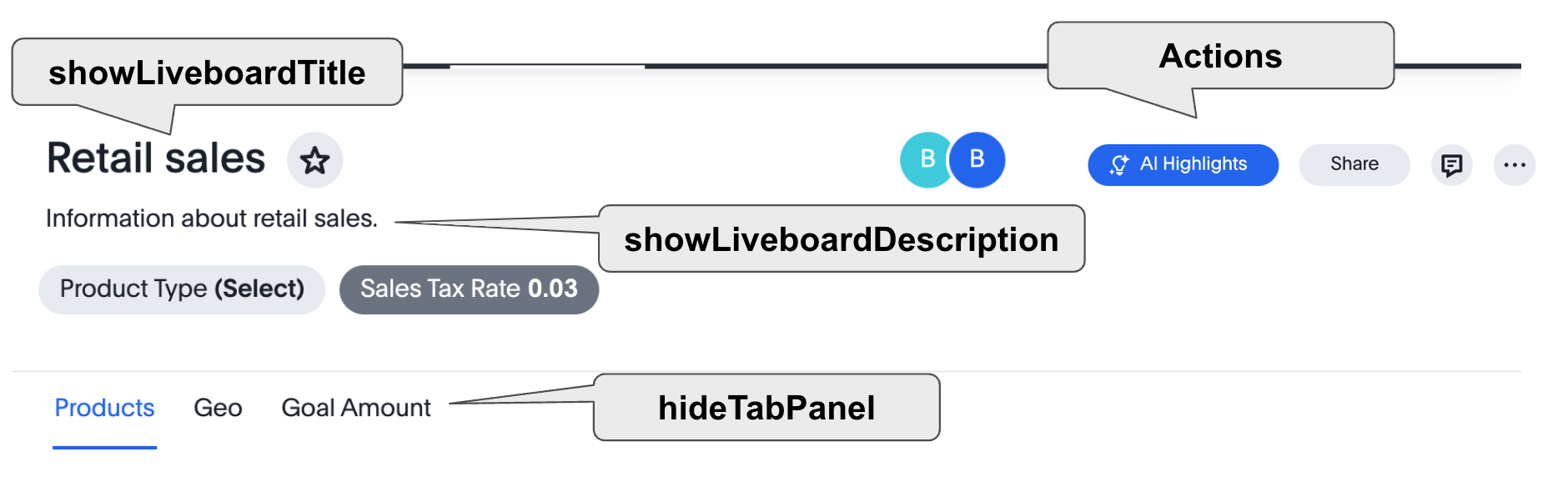
The hideLiveboardHeader
property removes the entire header area above the Liveboard, including filters and the overall Liveboard menu, which is a common pattern for "read-only' use cases or rebuilding your own menus and buttons using HostEvents.
The following constructor will disable the sticky header, while showing the Liveboard title, which would be hidden by default:
const liveboardEmbed = new LiveboardEmbed(document.getElementById('ts-embed'), {
frameParams: {
width: '100%',
height: '100%',
},
isLiveboardHeaderSticky : false,
showLiveboardTitle: true,
liveboardId: '<%=liveboardGUID%>',
});
Note
|
When |
Reduce visible tabs and visualizations🔗
visibleVizs
and visibleTabs
allow for limiting the experience for certain users from a Liveboard with many more elements.
For example, a template Liveboard with many different KPIs could be reduced down to a smaller set by giving a user an interface to select the particular visualizations to show, storing their selections, and using that saved set of visualization GUIDs as the array for visibleVizs
on page load (there is an equivalent HostEvent called SetVisibleVizs
to make an update after the Liveboard has loaded).
Redefine Liveboard breakpoint widths🔗
When turned on, the enable2ColumnLayout
allows for customising the Liveboard breakpoint width for embedded users.
The current 12 column layout changes to 2 columns per row at 1024px, and to 1 column per row layout at 630px in the new Liveboard experience. Once enabled, these breakpoints would apply to all Liveboards in the ThoughtSpot instance, and cannot be set only for individual Liveboards.
These breakpoint widths are customisable for the embedded customers. Contact ThoughtSpot support for assistance with customisation.
Liveboard experience🔗
Starting from 10.1.0.cl, the classic experience for liveboards has been deprecated.
Liveboard tabs🔗
The new Liveboard experience allows you to organize your visualizations into tabs. Liveboard tabs allow you to logically group visualization into specific categories and allow users to access them easily.
To create, edit, or move visualizations to a tab, you require edit access to a Liveboard.
-
To add a tab, click Edit and then click Add tab on the Liveboard page.
-
To add a visualization to a tab on a Liveboard, click Move to tab from the More
menu.
You can also pin a visualization to a Liveboard tab using the Pin action on the Answer page.
Set a tab as an active tab🔗
By default, the first tab created on a Liveboard is set as the home tab. You can set any tab as an active tab using the activeTabId
property in the Visual Embed SDK as shown in the example here:
const liveboardEmbed = new LiveboardEmbed(document.getElementById('ts-embed'), {
frameParams: {
width: '100%',
height: '100%',
},
liveboardId: "d7a5a08e-a1f7-4850-aeb7-0764692855b8",
activeTabId: "05406350-44ce-488e-abc5-5e8cdd65cf3c",
});
Note
|
The |
Filters🔗
To view specific data across the tables and charts on a Liveboard, users can apply filters in the embedded view. You can apply filters before embedding a Liveboard or apply runtime filters via Visual Embed SDK. Embedding application users can also apply filters across visualizations using the cross-filter feature.
The Visual Embed SDK provides several types of host events to trigger an action to get or update filters:
Date filters🔗
For DATE
and DATE_TIME
data types, you must provide the date and time values in the Epoch time format when applying or updating runtime filters via SDK or REST API.
However, when updating filters using HostEvent.UpdateFilters
, you must specify the date filter type
. You can use one of the following values for type
:
-
EXACT_DATE
Examples:2023-07-31
,2023/07/31
-
EXACT_DATE_RANGE
Specify the start date and end date in thevalues
array. Ensure that the start date is lower than the end date.
Example:"2023-01-31","2023-03-31"
. -
EXACT_DATE_TIME
Example:2023/07/31 23:59:59
-
EXACT_TIME
Example:10:10
-
LAST_N_Period
Example:Last 2 week
-
LAST_PERIOD
Example:Last week
-
MONTH_ONLY
Example:July
-
MONTH_YEAR
Specify the month and year in thevalues
array.
Example:"July","2023"
. -
YEAR_ONLY
Example:2023
-
NEXT_N_PERIOD
Example:Next 7 week
-
NEXT_PERIOD
Example:Next week
-
QUARTER_YEAR
Example:"Q1","2023"
-
THIS_PERIOD
Example:This week
-
PERIOD_TO_DATE
Example:month to date
-
YESTERDAY
-
TODAY
-
TOMORROW
HostEvent.UpdateFilters examples🔗
liveboardEmbed.trigger(HostEvent.UpdateFilters, {
filter: {
column: "date",
oper: "EQ",
values: ["July","2023"],
type: "MONTH_YEAR"
}
});
liveboardEmbed.trigger(HostEvent.UpdateFilters, {
filter: {
column: "date",
operator: "BW_INC",
values: [
"2023-01-31","2023-03-31"
],
type: "EXACT_DATE_RANGE"
}
});
Note tiles🔗
You can add a Liveboard Note tile with custom text, images, and links on an embedded Liveboard.
-
Only users with edit access to a Liveboard can add a Note tile.
-
Users require
Can upload data
privilege to upload an image to the note tile. -
If you are adding links and images from an external site, or embedding multimedia or web page in an iFrame, make sure the URLs are added to CORS and CSP allowlists. For more information, see Security settings.
Commenting on Liveboards🔗
Users on a non-embedded ThoughtSpot application instance can add comments, reply to comments, or subscribe to comment threads on a Liveboard. However, if the Liveboard is embedded in another application, the comment icon will not be visible to the embedded application users regardless of their access privileges.
Additional resources🔗
-
For information about runtime overrides, see Runtime filters and Runtime Parameter overrides.
-
For code examples, see Code samples.
-
For more information about the SDK APIs and attributes, see Visual Embed SDK Reference Guide.