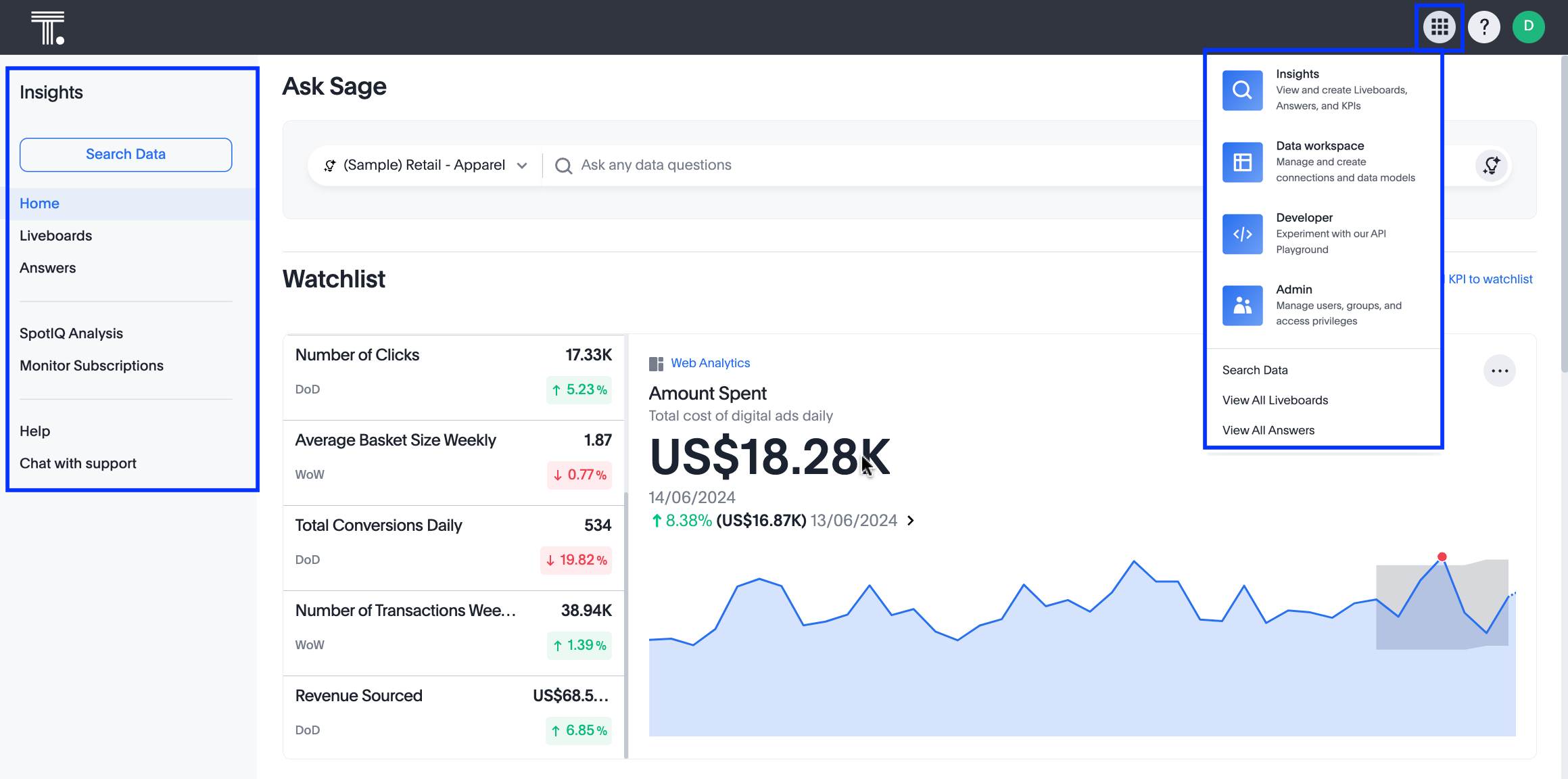
Customize full application embedding
- UI and navigation experience
- Choose the default page to load
- Search experience in full app embed
- Customize navigation controls
- Customize Home page modules (New experience)
- Detect changes in the currently loaded page
- Navigate using a custom action
- CSS customization and hiding page elements
- Additional resources
The AppEmbed component embeds the entire ThoughtSpot application experience within another page.
UI and navigation experienceπ
The ThoughtSpot UI and navigation experience is available in two modes:
-
Classic experience (default)
-
New navigation and Home page experience Early Access
New navigation and Home page experienceπ
In the new navigation and Home page experience, the app selector appears on the header bar instead of the top navigation menu. The app selector consists of different persona-based contextual elements called apps. On clicking an app from the application, the page corresponding to that app opens. Each application module has a separate left navigation panel.
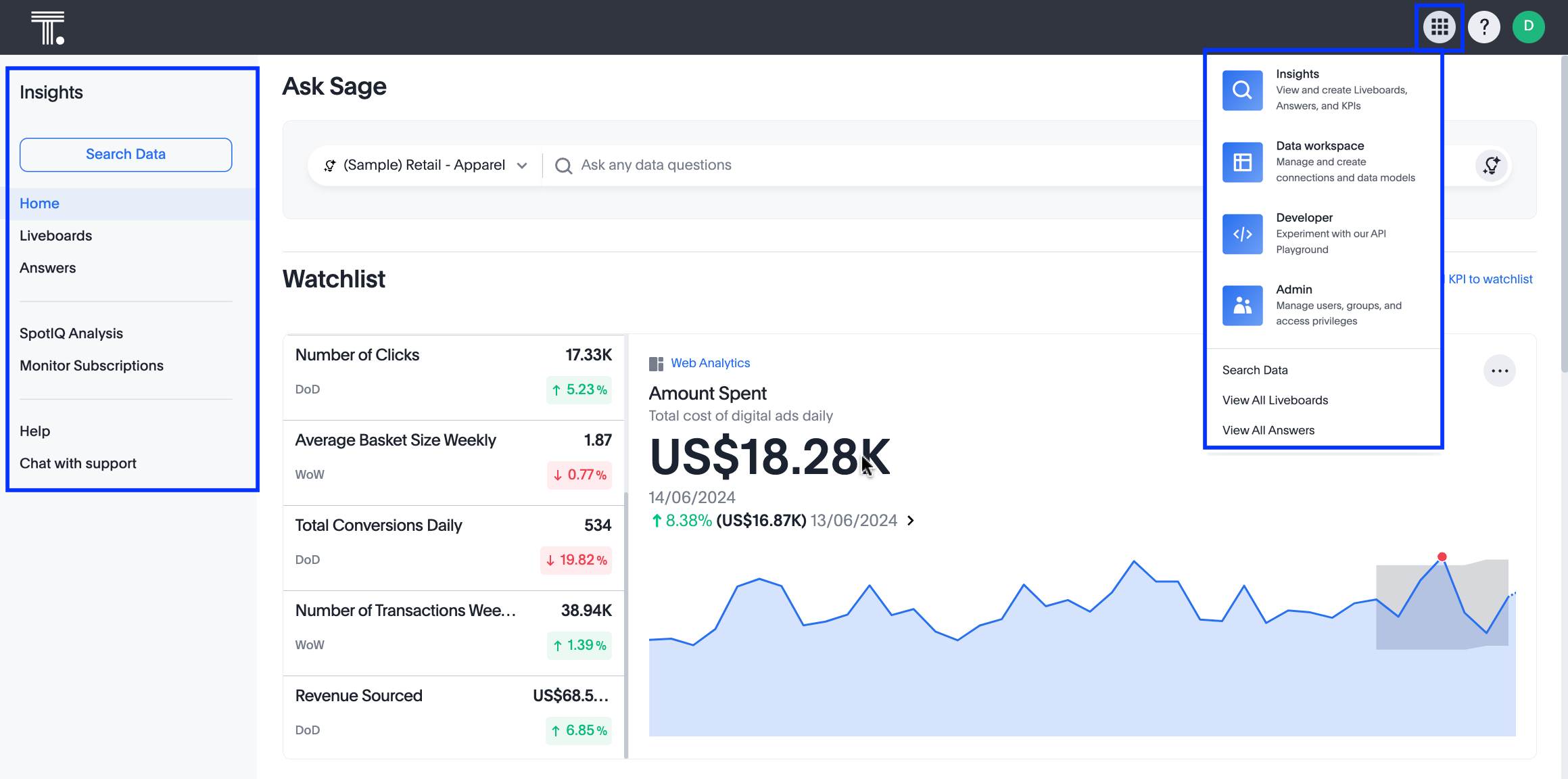
The new navigation and Home page experience introduces the following changes:
Classic experience | New navigation and Home page experience | |
---|---|---|
Navigation | Top navigation menu with the following buttons:
| App selector
|
Home page experience | In the classic experience mode, the Home page shows the Natural Language Search panel, a list of Answers and Liveboards, and trending charts. | The Insights page in new experience mode shows a customizable home page with features such as Natural Language Search panel, watchlist, favorites, a library of Answers and Liveboards, trending charts, and more. With the new left-hand navigation, users can navigate to your Liveboards, Answers, SpotIQ analysis, and Monitor subscriptions. |
Application page URLs |
|
|
Liveboards and Answers | In the classic experience mode, users can use All, Yours, and Favorites tabs to filter the Liveboards and Answers list | In new experience, the Liveboard and Answers list page provides filters for each column. For example, to view their favorite Liveboards, users can click the star icon in the column head and apply a filter to show only their starred (favorite) Liveboards. Similarly, users can filter the list by author to view only their Liveboards or Answers. |
Enable new experience mode for ThoughtSpot embeddingπ
By default, the new navigation and home page experience is turned off on ThoughtSpot embedding applications. To enable the new experience mode for embedding application users, set modularHomeExperience
to true
in the AppEmbed
component.
const embed = new AppEmbed("#embed", {
pageId: Page.Home,
modularHomeExperience: true,
frameParams: {
height: '100%',
width: '100%'
}
});
Choose the default page to loadπ
When embedding the full app, you can use either pageId
or path
parameter to specify the page to load when the embedded component loads. If both path
and pageId
properties are defined, the path
definition takes precedence.
pageIdπ
The pageId
parameter of the AppEmbed
parameters object lets you specify the ThoughtSpot page in the Page
enumeration that the AppEmbed component loads to. Valid values for this attribute are:
-
Page.Home
for the ThoughtSpot Home page -
Page.Search
for the ThoughtSpot Search page -
Page.Answers
for the Answers page -
Page.Liveboards
for the Liveboards page -
Page.Data
for the Data page -
Page.SpotIQ
for the SpotIQ analyses page
const embed = new AppEmbed("#embed", {
pageId: Page.Liveboards,
showPrimaryNavbar: false,
frameParams: {
height: '100%',
width: '100%'
}
});
pathπ
The URL path of the ThoughtSpot application page that you want your embed application users to navigate to.
const embed = new AppEmbed("#embed", {
path: 'pinboard/96a1cf0b-a159-4cc8-8af4-1a297c492ff9',
frameParams: {
height: '100%',
width: '100%'
}
});
The following examples show valid strings for path
:
Page | Classic experience | New navigation and Home page experience |
---|---|---|
Answers |
|
|
Saved Answer |
|
|
Liveboards |
|
|
Liveboard |
|
|
SpotIQ analysis list |
|
|
SpotIQ analysis page |
|
|
Data |
|
|
Worksheet, tables, views |
|
|
Monitor |
|
|
navigateToPage()π
The AppEmbed
object has a method called navigateToPage()
that will switch the currently loaded page in the ThoughtSpot embedded application. The navigateToPage()
method accepts the values that work for pageId
or path
parameters.
The new navigation menu should call navigateToPage
for the various pages you want to provide access to:
embed.navigateToPage(Page.Answers);
// with noReload option
embed.navigateToPage(Page.Answers, true);
history.back()π
Page changes within the AppEmbed
component register as part of the embedding appβs history to the web browser.
The standard JavaScript history.back()
function will cause the AppEmbed
component to go to the previously loaded page up until the very first ThoughtSpot page loaded within the component.
Search experience in full app embedπ
The Home page search experience varies based on the settings on your instance. On instances running 10.1.0.cl or lower, the Search interface on the Home page provides a combined view of Natural Language Search and Object Search. On instances running 10.3.0.cl and later, the Home page search experience is split into separate components.
-
If your instance was upgraded from 10.1.0.cl to 10.5.0.cl, Natural Language Search will be set as the default search experience for the Home page and the split search experience will be turned off by default. For applications embedding full ThoughtSpot experience, the
isUnifiedSearchExperienceEnabled
property will be set totrue
in the SDK. Your users can continue to use the unified experience until its deprecation. Developers can choose to disable the unified search experience and customize the Home page search experience for their users if required. -
If your instance was upgraded from 10.3.0.cl or 10.4.0.cl to 10.5.0.cl or later, the split search experience will be enabled by default and the
isUnifiedSearchExperienceEnabled
property will be set tofalse
in the SDK. As a result of this change, Object Search will be set as the default experience for the Home page in full application embedding. To enable AI Search for your embed application users, use one of the following options:-
Switch to AI search by setting
homePageSearchBarMode
toaiAnswer
in the SDK. -
Create a Natural Language Search page using SageEmbed and build a navigation to this page from your embedding application.
-
The following table lists the search components supported in full application embed and the configuration settings required for these components:
Type | Description |
---|---|
Object Search | Allows finding popular Liveboards and Answers from the recommended suggestions. On instances running 10.1.0.cl or lower, the Home page provides a combined interface with Object Search and Natural Language Search. On instances running 10.3.0.cl or later, with split search experience enabled, the Object Search will be the default search experience on the Home page. The Object Search bar also appears on the top navigation bar if the top navigation bar visibility is enabled ( |
Natural Language Search | Allows searching a data source using a natural language query string and get AI-generated Answers.
On instances running 10.3.0.cl or earlier, with split search experience disabled, Natural Language Search will be available along with Object Search on the Home page. However, on instances running 10.3.0.cl or later, split search is enabled by default, and due to this, the Home page will not show Natural Language Search as the default search experience. To enable Natural Language Search for embed users, set For more information, see Enable AI Search. |
SpotterBeta | In addition to AI Search capabilities, Spotter provides a conversation interface for queries and follow-up questions. For more information, see Enable AI Search with Spotter. |
Search data | Allows searching a data source using keywords and search tokens. This experience is available if you have set the |
Customize Home page search experienceπ
Developers can customize the Search experience by setting the homePageSearchBarMode
property in the SDK to a desired value:
-
objectSearch
(default)
Displays Object Search bar on the Home page. -
aiAnswer
Displays the search bar for Natural Language Search -
none
Hides the Search bar on the Home page. Note that it only hides the Search bar on the Home page and doesnβt affect the Object Search bar visibility on the top navigation bar.
Enable AI Searchπ
To set AI Search as the default search experience on the Home page, use the settings shown in the following examples.
New Home page experienceπ
const embed = new AppEmbed("#embed", {
modularHomeExperience: true,
homePageSearchBarMode: "aiAnswer",
});
- Home page search experience
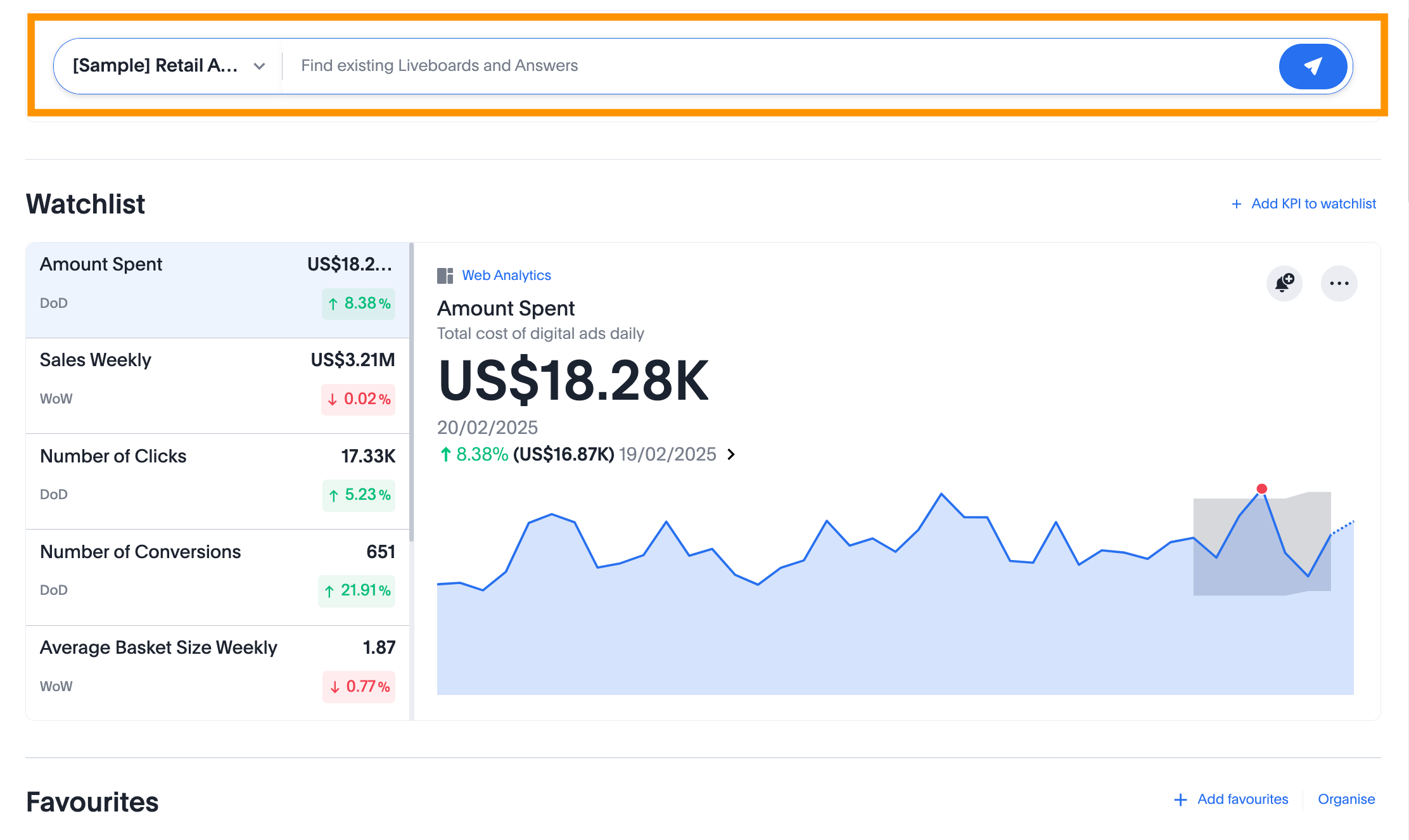
- AI Search page
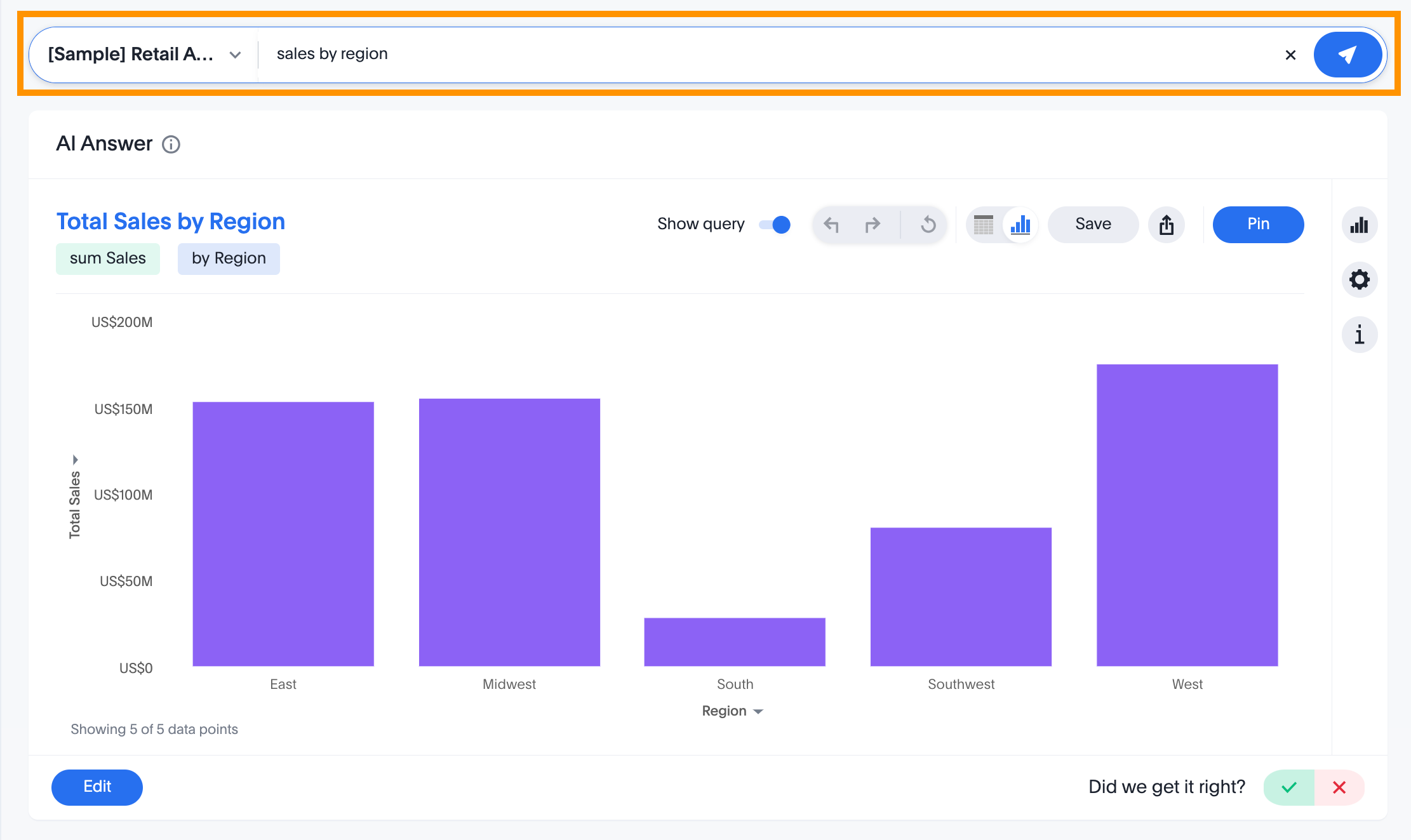
Home page classic experienceπ
const embed = new AppEmbed("#embed", {
homePageSearchBarMode: "aiAnswer",
});
- Home page search experience
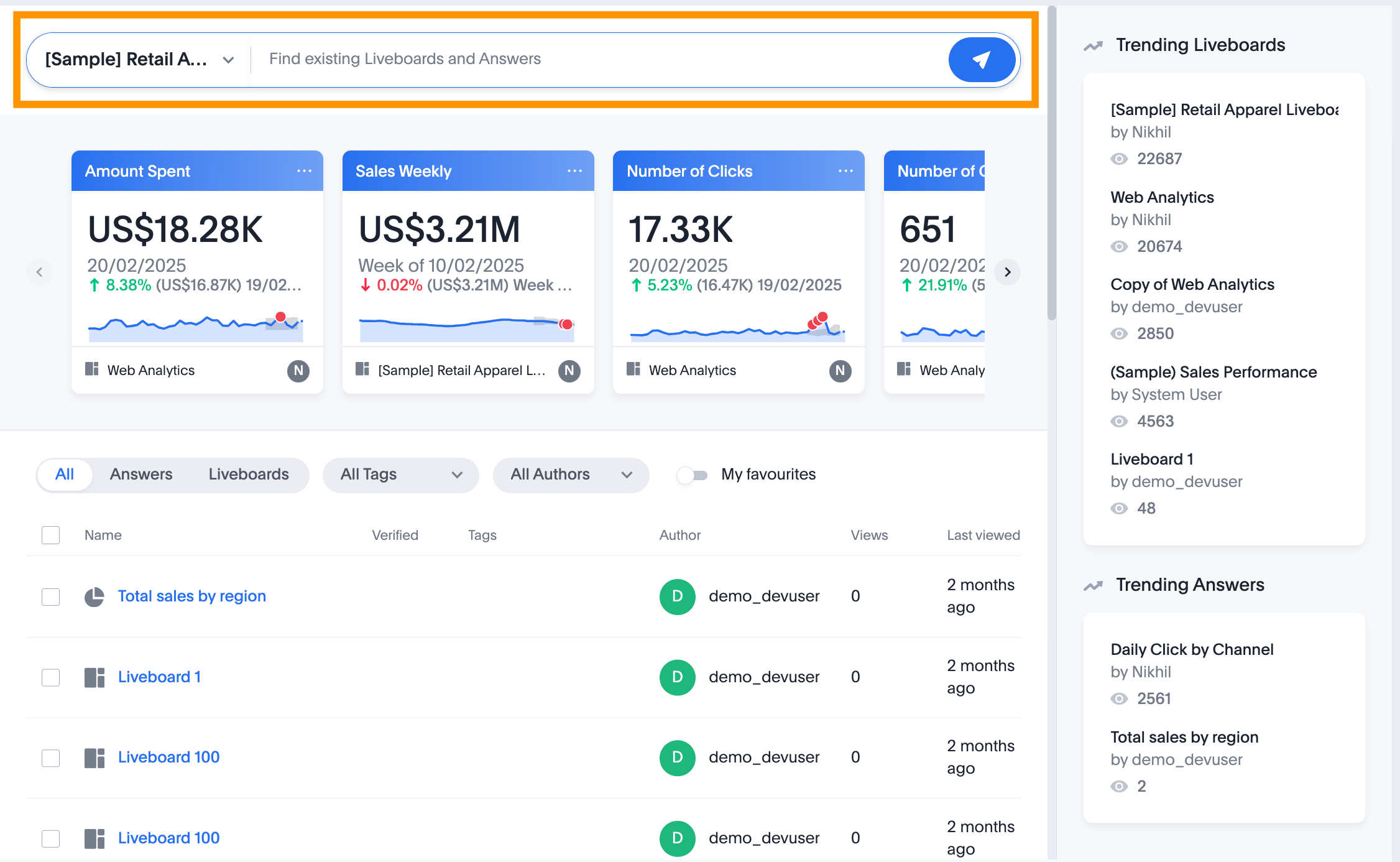
- AI Search page
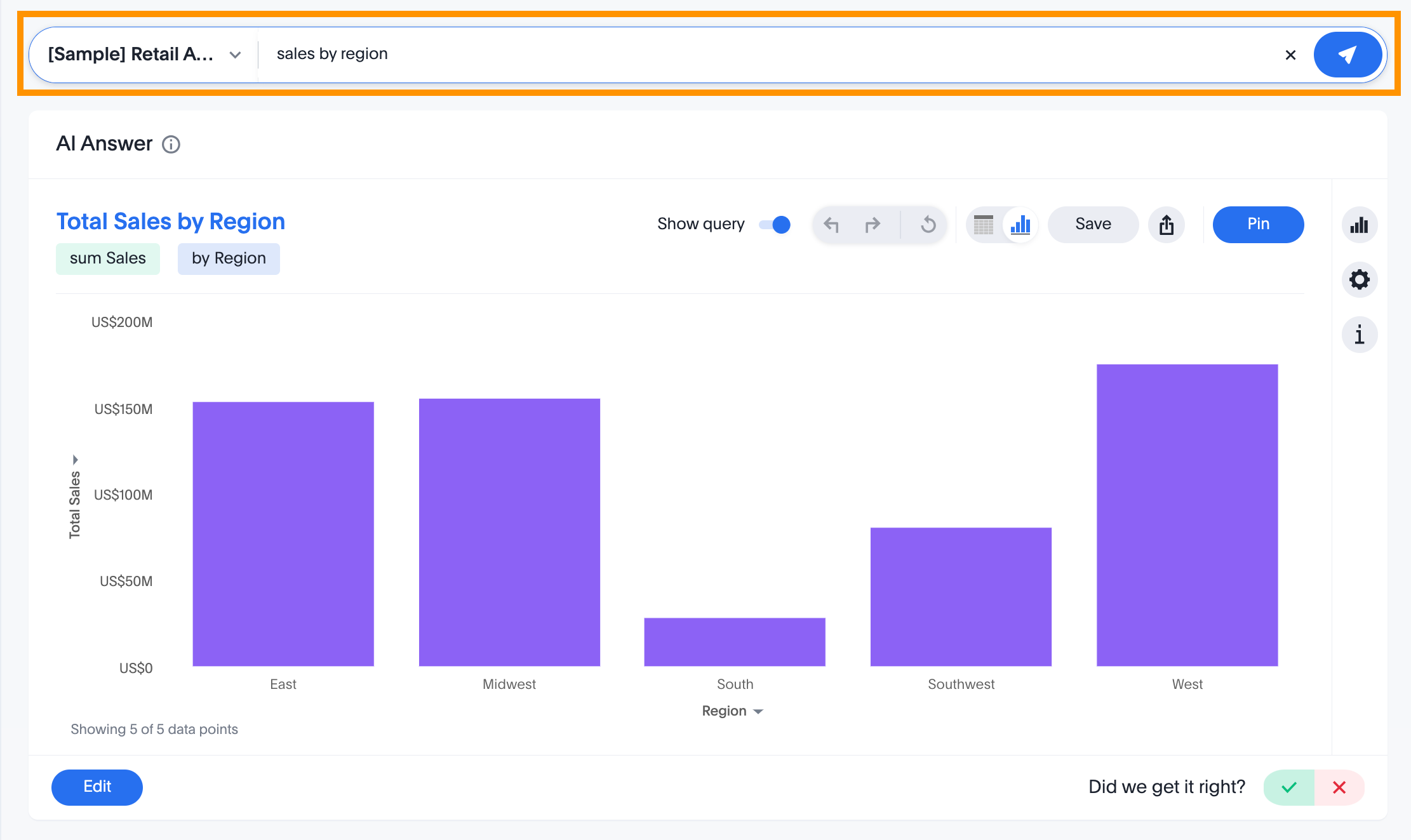
Enable AI Search with Spotterπ
To set Spotter as the default search experience on the Home page, use the settings shown in the following examples.
New Home page experienceπ
const embed = new AppEmbed("#embed", {
modularHomeExperience: true,
isUnifiedSearchExperienceEnabled: "false",
homePageSearchBarMode: "aiAnswer",
});
- Home page search experience
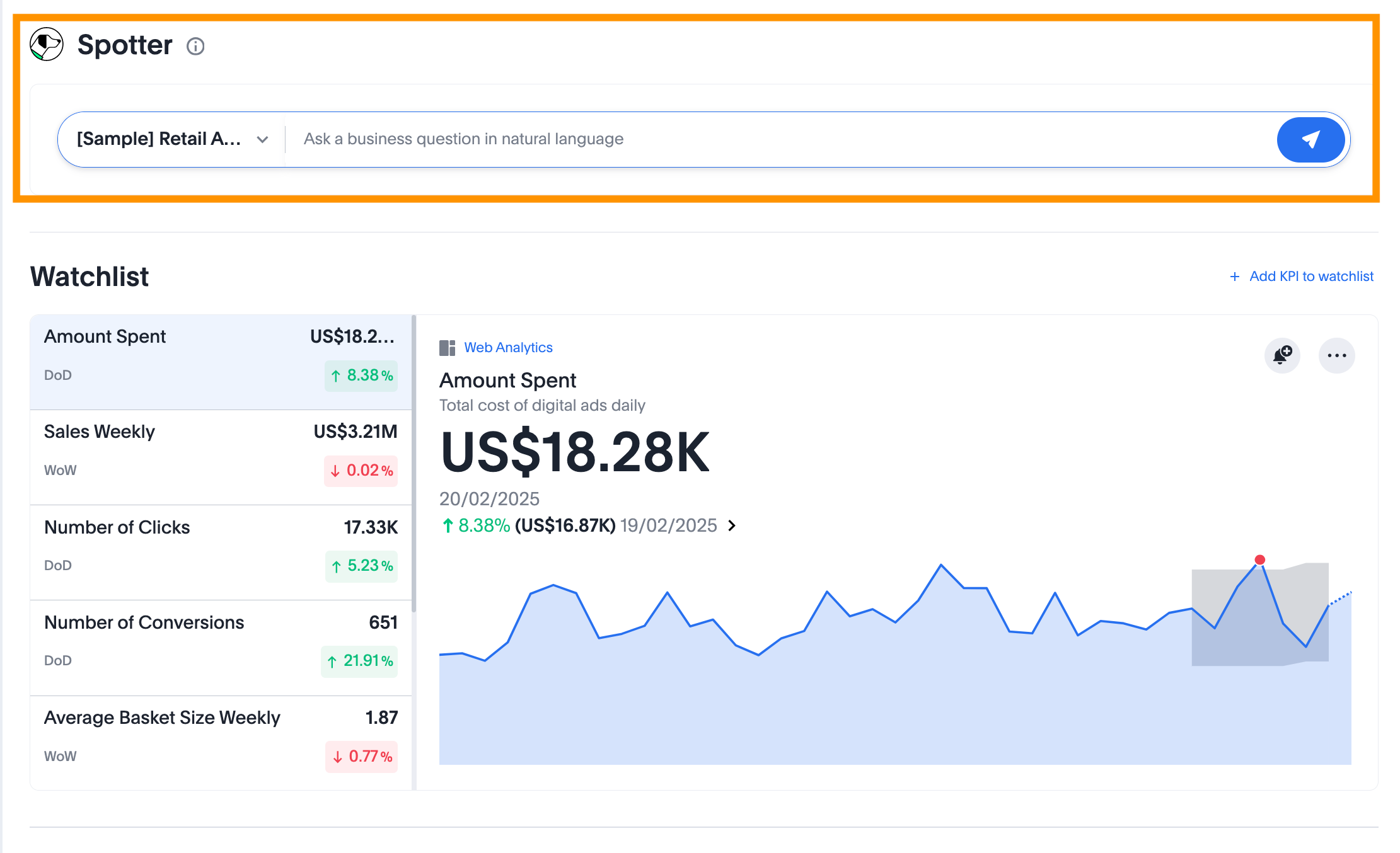
- Spotter page
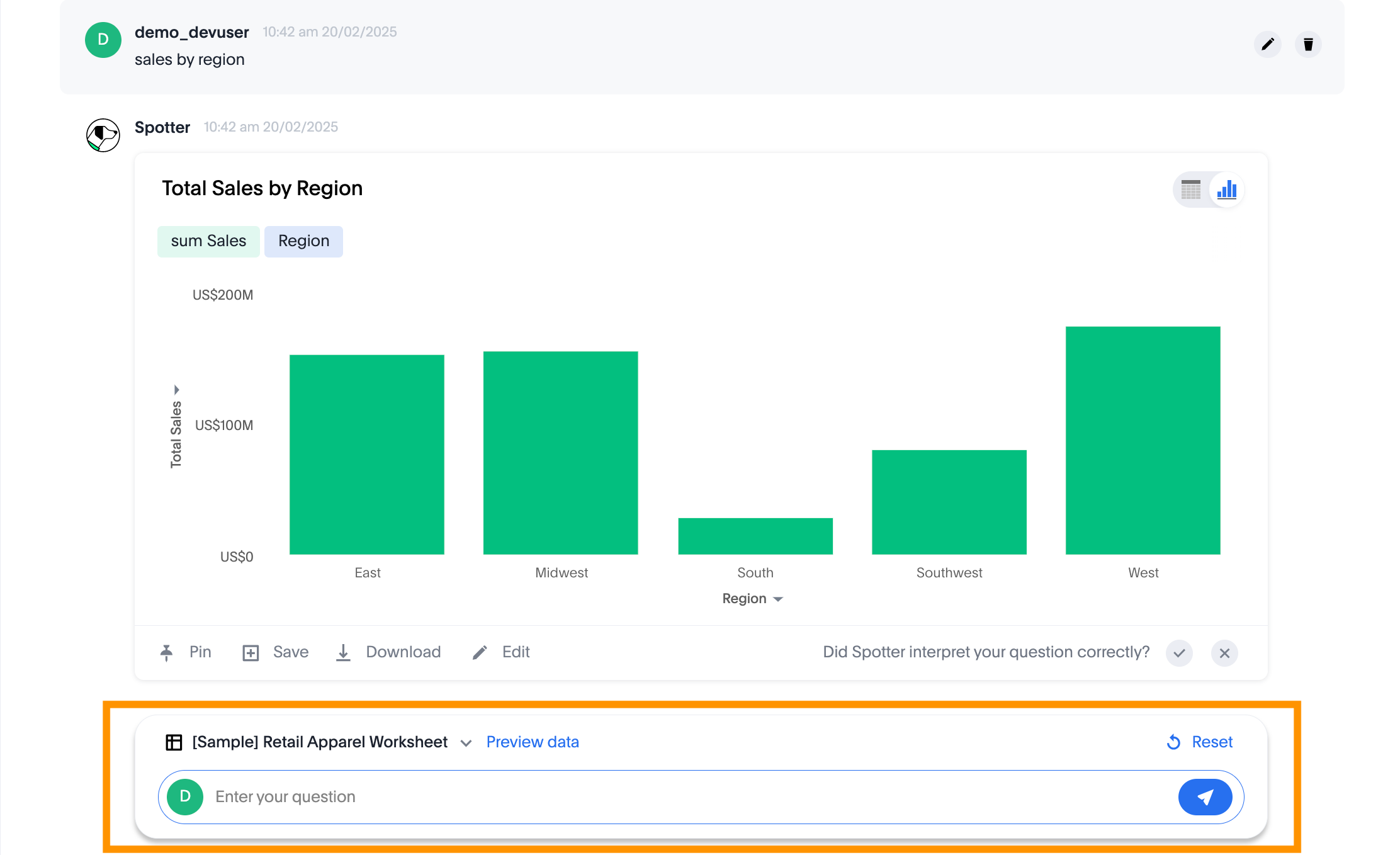
Home page classic experienceπ
const embed = new AppEmbed("#embed", {
isUnifiedSearchExperienceEnabled: "false",
homePageSearchBarMode: "aiAnswer",
});
- Home page search experience
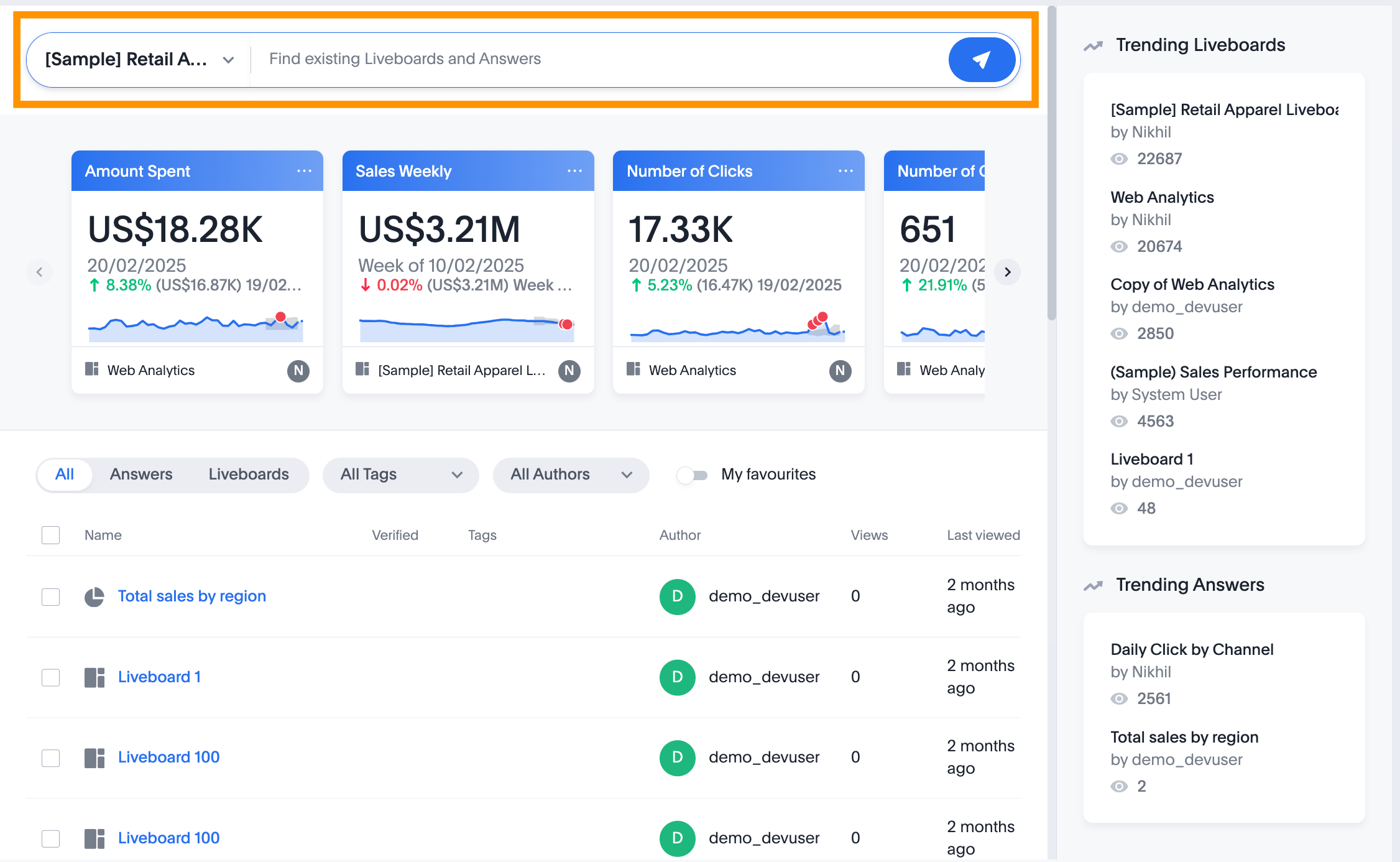
- Spotter page
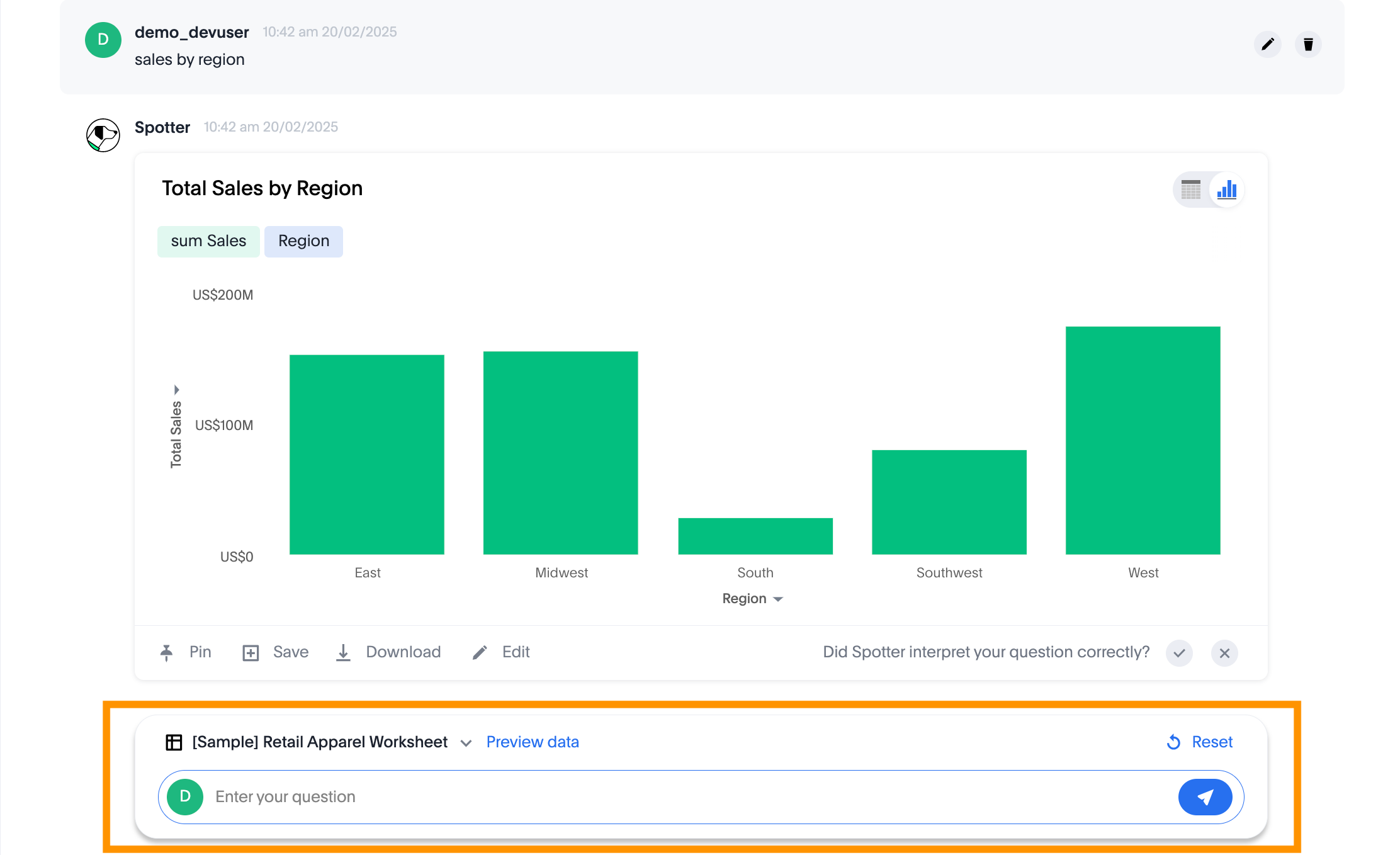
Customize navigation controlsπ
The AppEmbed
package in the Visual Embed SDK provides several parameters to hide or customize navigation controls.
The top navigation menu bar (classic experience), app selector (New experience), and left navigation panel on the home page (New experience) are hidden by default in the embedded view. To show these elements in the embedded view, set
showPrimaryNavbar
to true
. If the navigation panel is visible in the embedded view, you can use the following parameters in the AppEmbed
component for additional customization:
-
hideOrgSwitcher
Hides the Orgs drop-down. Applicable to only Orgs-enabled clusters. -
hideApplicationSwitcher
Hides the app selector. The app selector is available only in the new navigation and Home page experience mode.
-
disableProfileAndHelp
-
To hide help and profile icons (Classic experience)
-
To hide help and profile icons, Help and Chat with Support menu options on the Home page (New Experience).
-
Customize the left navigation panel on Home page (New experience)π
If the new navigation and Home page experience is enabled and showPrimaryNavbar
to true
, the home page displays a navigation panel on the left side of the Insights page. The panel consists of menu items such as Answers, Liveboards, SpotIQ Analysis, Monitor Subscriptions, and so on.
To hide the left navigation panel in the embedded view, set hideHomepageLeftNav
to true
.
const embed = new AppEmbed("#embed", {
modularHomeExperience: true,
showPrimaryNavbar: true,
hideApplicationSwitcher: true,
hideHomepageLeftNav: true,
disableProfileAndHelp: true,
});
If you donβt want to hide the left navigation panel, but show only a select few menu items, use hiddenHomeLeftNavItems
array.
const embed = new AppEmbed("#embed", {
modularHomeExperience: true,
showPrimaryNavbar: true,
hiddenHomeLeftNavItems: [HomeLeftNavItem.Home,HomeLeftNavItem.Liveboards],
});
Customize Home page modules (New experience)π
If the new navigation and Home page experience is enabled on your ThoughtSpot instance, the Home page shows modules such as watchlist, favorites, a library of Answers and Liveboards, trending charts and more. To customize these modules and the Home page experience, use the hiddenHomepageModules
array.
const embed = new AppEmbed("#embed", {
modularHomeExperience: true,
hiddenHomepageModules : [HomepageModule.Learning,HomepageModule.MyLibrary]
});
To reorder Home page modules, use the reorderedHomepageModules
array.
const embed = new AppEmbed("#embed", {
modularHomeExperience: true,
reorderedHomepageModules:[HomepageModule.Search,HomepageModule.Favorite,HomepageModule.Trending]
});
Detect changes in the currently loaded pageπ
Various actions the user takes within the embedded ThoughtSpot application may cause navigation within ThoughtSpot.
The embedding web application can listen for the EmbedEvent.RouteChange
event by attaching an event listener to the AppEmbed
object. The response has a currentPath
property which is the path after the ThoughtSpot domain, for example:
pinboard/96a1cf0b-a159-4cc8-8af4-1a297c492ff9
To parse the currentPath
into varying useful components, this tsAppState
object code can be created in the global scope for use by any other web application code:
// Simple global object to handle details about what is visible in the AppEmbed component at a given moment
let tsAppState = {
currentPath: startPath,
currentDatasources: [], // Can be set later when detected from TML or other events
// return back what is being viewed at the moment, in the form that will translate to the pageId property if captialized, or path property if not
get pageType() {
if (this.currentPath.includes('/saved-answer/')){
return 'answer';
}
else if (this.currentPath.includes('/pinboard/')){
return 'liveboard';
}
/*
* Others are meant to match the exact pageId from SDK
*/
else if(this.currentPath.includes('/answer/')){
return 'Search';
}
else if(this.currentPath.includes('/answers')){
return 'Answers';
}
else if (this.currentPath.includes('/pinboards')){
return 'Liveboards';
}
else if(this.currentPath.includes('/insights')){
return 'SpotIQ';
}
else if(this.currentPath.includes('/monitor')){
return 'Monitor';
}
else if(this.currentPath.includes('/data')){
return 'Data';
}
else {
return 'Home';
}
},
// If viewing an Answer or Liveboard, returns the GUID of that object from the parsed URL
get objectId() {
let pathParts = this.currentPath.split('/');
// '/saved-answer/' is path for Answers (vs. /answer/)
if (this.currentPath.includes('/saved-answer/')){
answerGUID = pathParts[2];
return pathParts[2];
}
// '/pinboard/' is path for saved Liveboards
else if (this.currentPath.includes('/pinboard/')){
let pathParts = this.currentPath.split('/');
// May need adjustment for tabbed views to add in current Tab
liveboardGUID = pathParts[2];
return pathParts[2];
}
else{
return null;
}
}
}
The following example shows the event listener code updating the global tsAppState
object above whenever there is a change within the embedded ThoughtSpot app:
embed.on(EmbedEvent.RouteChange, (response) => {
// console.log("RouteChange fires");
// console.log(response);
// tsAppState object has currentPath property, which allows its other methods to parse out pageId, object type, GUIDs etc.
tsAppState.currentPath = response.data.currentPath;
console.log("TS App page is now: ", tsAppState.currentPath);
// Update elements within your web application based on the new state of ThoughtSpot (adjust menu selections, etc.)
})
Navigate using a custom actionπ
To add a custom action for in-app navigation, follow these steps:
-
Define the navigation path
In this example, the view-report action on a Liveboard page calls the navigateTo
method to open a specific saved Answer page when a user clicks the View report button in the embedded app.
appEmbed.on(EmbedEvent.CustomAction, async (payload: any) => {
if (payload.payload.id === 'view-report') {
appEmbed.navigateToPage(
'saved-answer/3da14030-11e4-42b2-8e56-5ee042a8de9e'
);
}
})
If you want to navigate to a specific application page without initiating a reload, you can set the noReload
attribute to true
as shown here:
appEmbed.on(EmbedEvent.CustomAction, async (payload: any) => {
if (payload.payload.id === 'view-report') {
appEmbed.navigateToPage('saved-answer/3da14030-11e4-42b2-8e56-5ee042a8de9e', true);
}
})
CSS customization and hiding page elementsπ
CSS customization allows overriding the default styles from the ThoughtSpot application, including the application pages.
If there is an element of a page that you dislike and cannot hide with any combination of other options in ThoughtSpot, you can often use CSS customization to target the element and apply either display: none;
, visibility: hidden;
or height: 0px;
and make it functionally disappear to the end user.
Specifying a direct element using the direct CSS selectors vs. the ThoughtSpot provided variables. To discover the appropriate selector, use the Inspect functionality of your browser to bring up the Elements portion of the browserβs Developer Tools, then look at the Styles information.
An example of using direct selectors in a file is available in the complete.css.
.bk-data-scope .left-pane .header-lt {
display: none !important;
visibility: hidden !important;
}
Direct selectors can also be declared using rules in the Visual Embed SDK code. This is useful for real-time testing, particularly in the Visual Embed SDK playground. Note the format for encoding CSS rules into the JavaScript object format used by for rules.