curl -X POST \ --url 'https://{ThoughtSpot-Host}/api/rest/2.0/auth/token/full' \ -H 'Accept: application/json'\ -H 'Content-Type: application/json' \ --data-raw '{ "username": "tsUserA", "password": "Guest@123!" "validity_time_in_sec": 86400 }'
Get started with REST API v2.0
Before you get started with REST API v2.0, visit the REST API v2.0 Playground to view the endpoints and try out the API requests.
Visit the API Playground🔗
To access the REST API v2.0 Playground, go to Develop > REST API > REST Playground v2.0.
If you are using the new experience, the Developer will be in the Application switcher .
The Playground allows you to make API calls, view the request and response workflows, and create and download code samples.
Set up your environment🔗
By default, your cluster URL is set as the base path for your API requests.
-
To allow a REST client to log in to ThoughtSpot using
username
andpassword
, create a local user account in ThoughtSpot. -
To use OAuth 2.0 authentication method, make sure your ThoughtSpot instance has the required configuration to support the OpenID Provider or IdP that authenticates your users.
-
To trusted authentication to authenticate REST clients, make sure Trusted authentication is enabled on your ThoughtSpot instance, and your authenticator service has access to the
secret key
.
Authorize your client🔗
To provide secure access to ThoughtSpot resources, the REST API v2.0 framework supports token-based authentication method. However, for local development or testing purposes, you can use basic authentication with userName
and password
.
To get a token that provides access to ThoughtSpot resources, you can send a POST
request to one of the following API endpoints:
-
/api/rest/2.0/auth/token/object
Provides a token to access a specific metadata object such as a Liveboard or saved Answer.
-
api/rest/2.0/auth/token/full
Provides full access to ThoughtSpot.
For example, to get a bearer token that grants full access to ThoughtSpot application, send the following attributes in the request body:
If the API request is successful, the server returns the access token in the response body.
{
"token": "{access-token}",
"creation_time_in_millis": 1675129264089,
"expiration_time_in_millis": 1675129564089,
"scope": {
"access_type": "FULL",
"org_id": 1,
"metadata_id": null
},
"valid_for_user_id": "59481331-ee53-42be-a548-bd87be6ddd4a",
"valid_for_username": "tsUserA"
}
Note
|
By default, the bearer token is valid for 300 seconds. You can configure the token expiry duration as per your requirement or request a new token for your API sessions. If you send an API request with an expired token, the server returns an error. |
Log in to ThoughtSpot🔗
To log in to ThoughtSpot, send a POST
request to the /api/rest/2.0/auth/session/login
API endpoint. In your API request, you can either specify your username
and password
or use your bearer token in the authorization header.
After a successful login, a session cookie is set for your subsequent API calls. REST clients accessing APIs in a non-browser environment must set session cookies in the request header when making an API call.
Make a test API call🔗
To make a test API call, send an API request to an API endpoint. For example, to get a list of Liveboard objects, send the following request:
curl -X POST \
--url 'https://{ThoughtSpot-host}/api/rest/2.0/metadata/search' \
-H 'Authorization: Bearer {access_token}' \
-H 'Accept: application/json' \
-H 'Content-Type: application/json' \
--data-raw '{
"metadata": [
{
"type": "LIVEBOARD"
}
]
}'
Request headers🔗
Each API call must include the following headers.
-
'Authorization: Bearer {access_token}'
The authorization header must include the OAuth token obtained from ThoughtSpot.
-
'Content-Type: application/json'
The header to indicate the content type for the request body.
-
'Accept: application/json'
The
Accept
header for API response format.
-
User-Agent
The
User-Agent
header is required for all requests. Most clients will add theUser-Agent
header automatically. However, when making API calls from code, especially.NET
, you must add theUser-Agent
header.The
User-Agent
can be any string; for example, you can set the header as'User-Agent: <browser>/<browser-version><os/platform>'
.
Request body🔗
The API calls require you to specify the GUID or name of the object as an identifier. For example, to get details of a user object, you can specify either the GUID or name of the user.
The API Playground indicates the required and optional parameters and provides information about the data type and allowed values. For example, to get a list of answers, you must specify the metadata type
as Answer
.
The following example shows the cURL request format to get a list of answers saved in the ThoughtSpot system.
curl -X POST \
--url 'https://{ThoughtSpot-host}/api/rest/2.0/metadata/search' \
-H 'Authorization: Bearer {access_token}'\
-H 'Accept: application/json'\
-H 'Content-Type: application/json' \
--data-raw '{
"metadata": [
{
"type": "ANSWER"
}
]
}
Data format🔗
The REST APIs allow you to send and receive data in JSON format. To embed this data in your application, you can import or extract the data from the JSON file.
API response🔗
A successful API call returns a response body or the 204 response code. The REST API v2.0 framework supports standard HTTP response codes to indicate the status of a request.
-
200
Indicates a successful operation. The API returns a response body.
-
204
Indicates a successful operation. The 204 response does not include a response body.
-
400
Indicates a bad request. You may have to modify the request before making another call.
-
401
Indicates an unauthorized request. Check if you have the required credentials and object access to send the API request.
-
403
Indicates forbidden access. Check your access privileges and user account status.
-
415
Indicates an unsupported media type. Check the media type specified in the
Content-Type
header. -
500
Indicates an internal server error. Check if the data format of the request is supported. Verify if the server is available and can process the request.
If an API call returns an error in the Playground, you can view the error details by navigating to root > error > message > debug in the error response body.
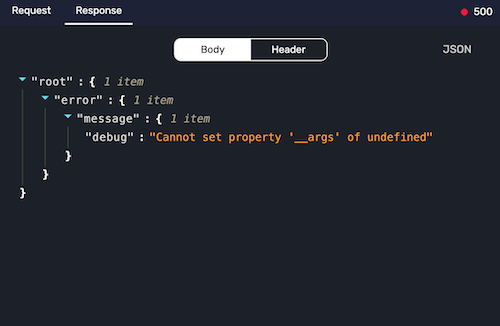
Date format in API response🔗
If Custom Calendar is not enabled on your instance, the API output shows the date and time data in Epoch time format.
[694252800,702115200,709974000,717922800,725875200,733651200,741510000,749458800,757411200,765187200,773046000,780994800,788947200,796723200,804582000,812530800,820483200,828345600,836204400,844153200,852105600,859881600,867740400,875689200,883641600,891417600,899276400,907225200]
If Custom Calendar is enabled on your instance, the data returned in the API response shows the date and time in the {"v":{"s":694252800}}
format.
[{"v":{"s":694252800}},{"v":{"s":702115200}},{"v":{"s":709974000}},{"v":{"s":717922800}},{"v":{"s":725875200}},{"v":{"s":733651200}},{"v":{"s":741510000}},{"v":{"s":749458800}},{"v":{"s":757411200}},{"v":{"s":765187200}},{"v":{"s":773046000}},{"v":{"s":780994800}},{"v":{"s":788947200}},{"v":{"s":796723200}},{"v":{"s":804582000}},{"v":{"s":812530800}},{"v":{"s":820483200}},{"v":{"s":828345600}},{"v":{"s":836204400}},{"v":{"s":844153200}},{"v":{"s":852105600}},{"v":{"s":859881600}},{"v":{"s":867740400}},{"v":{"s":875689200}},{"v":{"s":883641600}},{"v":{"s":891417600}},{"v":{"s":899276400}},{"v":{"s":907225200}}]
-
v
indicates value -
s
indicates the start date in Epoch time format -
e
indicates the end date in Epoch time format
If the API implementation in your environment is designed to use the date output in Epoch format, this change may break your current setup.
Additional resources🔗
-
See REST API tutorials for a comprehensive hands-on guide on REST API v2.0.
-
The thoughtspot_rest_api_v1 Python library includes a
TSRestApiV2
class that implements the v2.0 REST API. You can use this as a ready-made implementation for testing back-end REST API calls or as a pattern for implementing your own library in any other programming language.