import {
SearchBarEmbed,
AuthType,
init,
prefetch,
EmbedEvent,
HostEvent
}
from '@thoughtspot/visual-embed-sdk';
Embed ThoughtSpot search bar
If you want to embed just the ThoughtSpot search bar in applications such as Google Sheets, use the SearchBarEmbed
package in the Visual Embed SDK.
The SearchBarEmbed
package allows you to embed the following components:
-
Search bar
ThoughtSpot search bar that allows passing search tokens. The search bar is responsive and can be adjusted to fit the screen width of your web application or mobile app.
-
Get data button
The Get data button returns the search result as raw data. Your host application can use this data as a payload and render it in your app.
-
Data panel
The data sources panel allows users to choose the data sources to search from. The current version of the SDK doesnβt allow you to hide or collapse the data panel.
Import the SearchBarEmbed packageπ
Import the SearchBarEmbed
SDK library to your application environment:
npm
ES6
<script type = 'module'>
import {
SearchBarEmbed,
AuthType,
init,
prefetch,
EmbedEvent
}
from 'https://cdn.jsdelivr.net/npm/@thoughtspot/visual-embed-sdk/dist/tsembed.es.js';
Initialize the SDKπ
Initialize the SDK and define authentication attributes.
Create an instance of the SearchBarEmbed classπ
Create an instance of the SearchBarEmbed object and customize your search page view.
const searchBarEmbed = new SearchBarEmbed(document.getElementById('ts-embed'), {
frameParams: {
width: '100%',
height: '100%',
},
dataSources: ['<%=datasourceGUID%>'],
searchOptions: {
searchTokenString: '<TML-query-string>',
executeSearch: true,
},
});
For more information about the search bar embed object, classes, methods, interface properties, and enumeration members, see the following pages:
Register, handle, and trigger eventsπ
Register event listeners.
searchBarEmbed.on(EmbedEvent.Init, showLoader)
searchBarEmbed.on(EmbedEvent.Load, hideLoader)
searchBarEmbed.on(EmbedEvent.Data, payload => {
console.log('data', payload);
})
By default, ThoughtSpot returns 1000 rows of data for a given query when a user clicks the Get data button. To get more rows of data, you can subscribe to the GetDataClick
event and trigger a response payload from the search data API call.
The following example shows how to register the GetDataClick
event and trigger an API call:
const getQueryResult = async (query: string, sourceName: string) => {
const baseUrl = 'test.thoughspot.com';
const r = await fetch(`${baseUrl}/api/rest/2.0/searchdata`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
query_string: query,
logical_table_identifier: sourceName,
data_format: 'COMPACT',
record_size: 1000000,
}),
credentials: 'include',
});
const data = await r.json();
return {
colNames: data.contents[0].column_names,
rows: data.contents[0].data_rows,
};
};
tsembed.on(EmbedEvent.GetDataClick, ()=>{
tsembed.trigger(HostEvent.GetTML).then(async (data) => {
const query = data.answer.search_query;
if (!query) {
return;
}
const source = data.answer.tables[0].id;
const { colNames, rows } = await getQueryResult(query, source);
console.log(colNames, rows);
});
})
For more information about event types, see the following pages:
Render the embedded searchπ
searchBarEmbed.render();
Test your embeddingπ
Load the embedded object in your app. If the embedding is successful, you will see the following page:
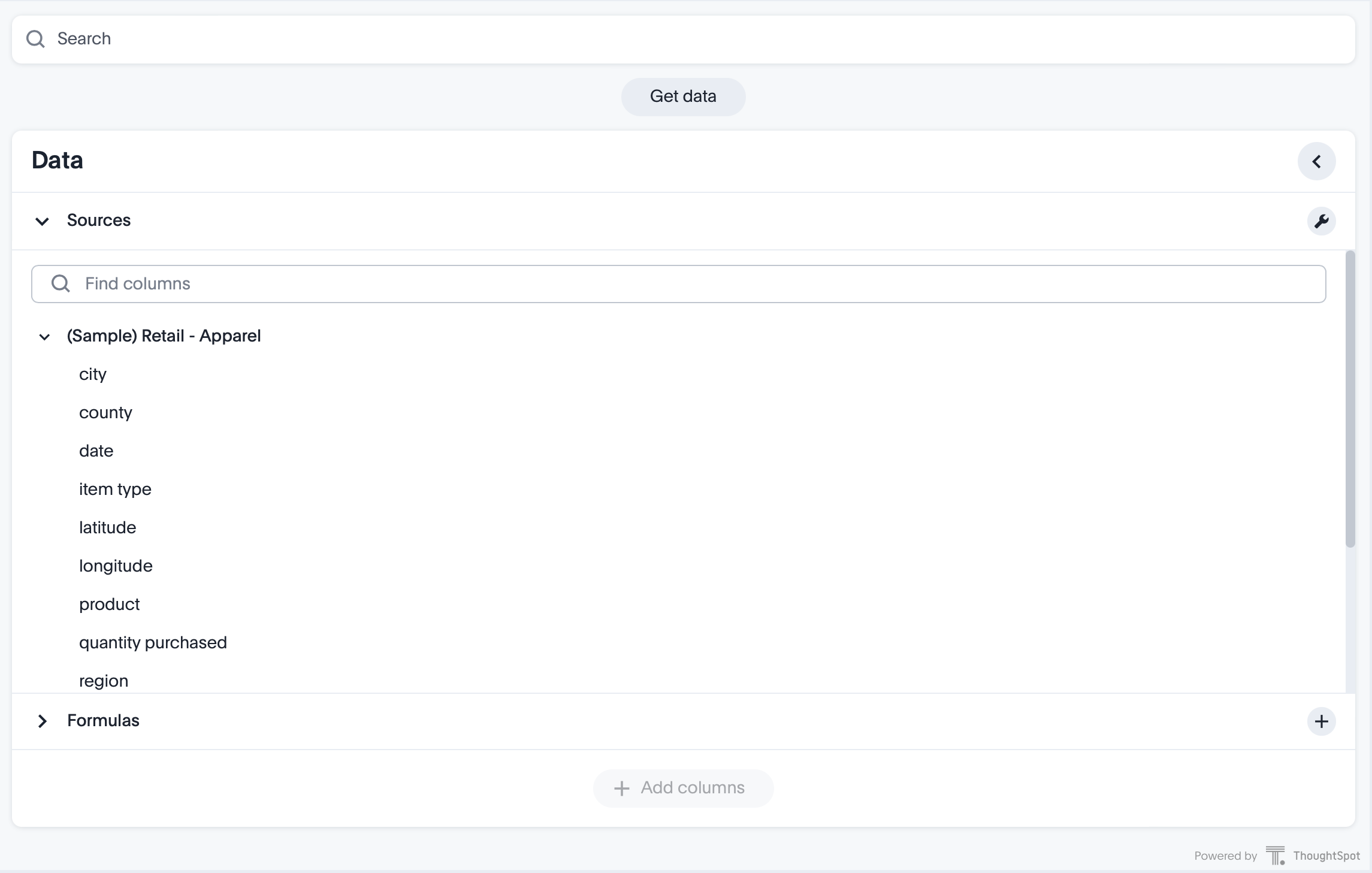
Run a search query and verify the results.