Content-Type: application/json Accept: application/json Authorization: Bearer {access-token}
What is a REST API?
Before you begin this lesson, check if you have done all initial activities described in the tutorial introduction.
All lessons are intended to be hands-on.
When you see a screenshot from ThoughtSpot or a code sample, please work along in your environment.
Overview of REST APIsπ
REST APIs are APIs that use web browser technology (HTTP) to send commands to a web server, which then does the requested actions and returns a response back to the commandβs sender.
HTTP is the communication protocol, so both sides can talk regardless of internal details.
Each specific command is called an endpoint, which is the URL of the command.
Any programming language or environment can use REST APIs with proper HTTP communication.
01 - REST API specificationsπ
While the HTTP communication method is standardized, the exact things to send and what will be received are different for every API.
The specifications of an API will describe each endpoint:
-
The endpoint itself (the full URL will be combination of server, API URL path, and the endpoint):
-
For example,
https://{subdomain}.thoughtspot.cloud/api/rest/2.0/metadata/search
-
-
The HTTP verb and headers to send
-
The body of a request
-
The expected response from the request, and any expected actions on the system caused by using the endpoint
Examples are often given using cURL which is the standard Linux command line tool for issuing direct HTTP commands.
ThoughtSpot REST API Playgroundπ
Every instance of ThoughtSpot has a REST API V2.0 Playground which is both the specification of the API and an interactive system for building and issuing API commands. The Playground is organized into topics, which compose the expandable areas of the left panel.
Click on any of the topics to expand the menu and see the individual endpoints that are available.
Most of the topics are self-explanatory. However, the following topics may not be obvious at first glance:
Authentication |
all session related endpoints including authentication token requests |
Metadata |
listings of objects that exist on the system, including the TML import and export endpoints |
Reports |
exports in a file format |
Security |
permissions and access control (sharing) |
Data |
exports in a JSON data format |
Once you click on an individual endpoint, you will see the specification for that endpoint in the middle area, and an example of using cURL to issue the request to the endpoint properly on the left:
02 - HTTP requestsπ
HTTP requests are composed of:
-
the endpoint URL
-
HTTP verb
-
headers
-
an optional body
HTTP methodsπ
There are 4 common request verbs:
GET |
read, no request body, can have parameters on the URL |
POST |
create, typically has a body |
PUT |
update, typically has a body |
DELETE |
delete, often only has a URL (but some systems use a body) |
Note
|
ThoughtSpot REST API V2.0 only uses GET and POST verbs. The full endpoint name includes the action the endpoint will take, rather than the same endpoint responding to different HTTP verbs. |
Any endpoint that ends in /search
is a GET
request for querying information.
HTTP headersπ
Headers describe aspects of the request, and are mandatory for any given endpoint.
ThoughtSpotβs V2.0 REST API typically requires the following headers:
The endpoints to request access tokens do not require the Authorization
header (they are the source of the tokens).
Endpoints that return something other than a JSON response do not use the Accept: application/json
header.
HTTP Bodyπ
Body is data sent with the request, that does not appear in the URL (is secure). The body will vary with each endpoint, and some endpoints do not require a body at all.
The body of all V2.0 REST API requests will be in JSON format.
You can copy the JSON request from the Playground and use directly in JavaScript or Typescript, or with very minimal modification in Python code.
03 - HTTP responsesπ
Each HTTP request will result in some action within the web server and then a response.
For GET requests, the only expected action is a response with some element. For example, the /search
endpoints within the V2.0 REST API are GET requests that return sets of information about the system.
Other types of requests cause a state change on the server, and then a response describing the new state after the action has completed.
HTTP response codes: successes and errorsπ
HTTP defines numeric response codes with designated meanings:
200 |
request completed successfully and returned a response |
204 |
request completed successfully, no response |
302 |
redirect |
4XX |
request failed due to known error |
500 |
request failed due to unknown server error |
Make sure you are testing for "success" rather than just 200
, because the V2.0 REST API does return both 200
and 204
depending on the endpoint.
The Playground has a specification area at the bottom of the middle area showing what to expect from the various possible responses:
JSON response formatπ
If there is a 200
success, the model of the response is available as a link:
This will take you to a description of the response structure, as well as JSON example of the core model part of the response:
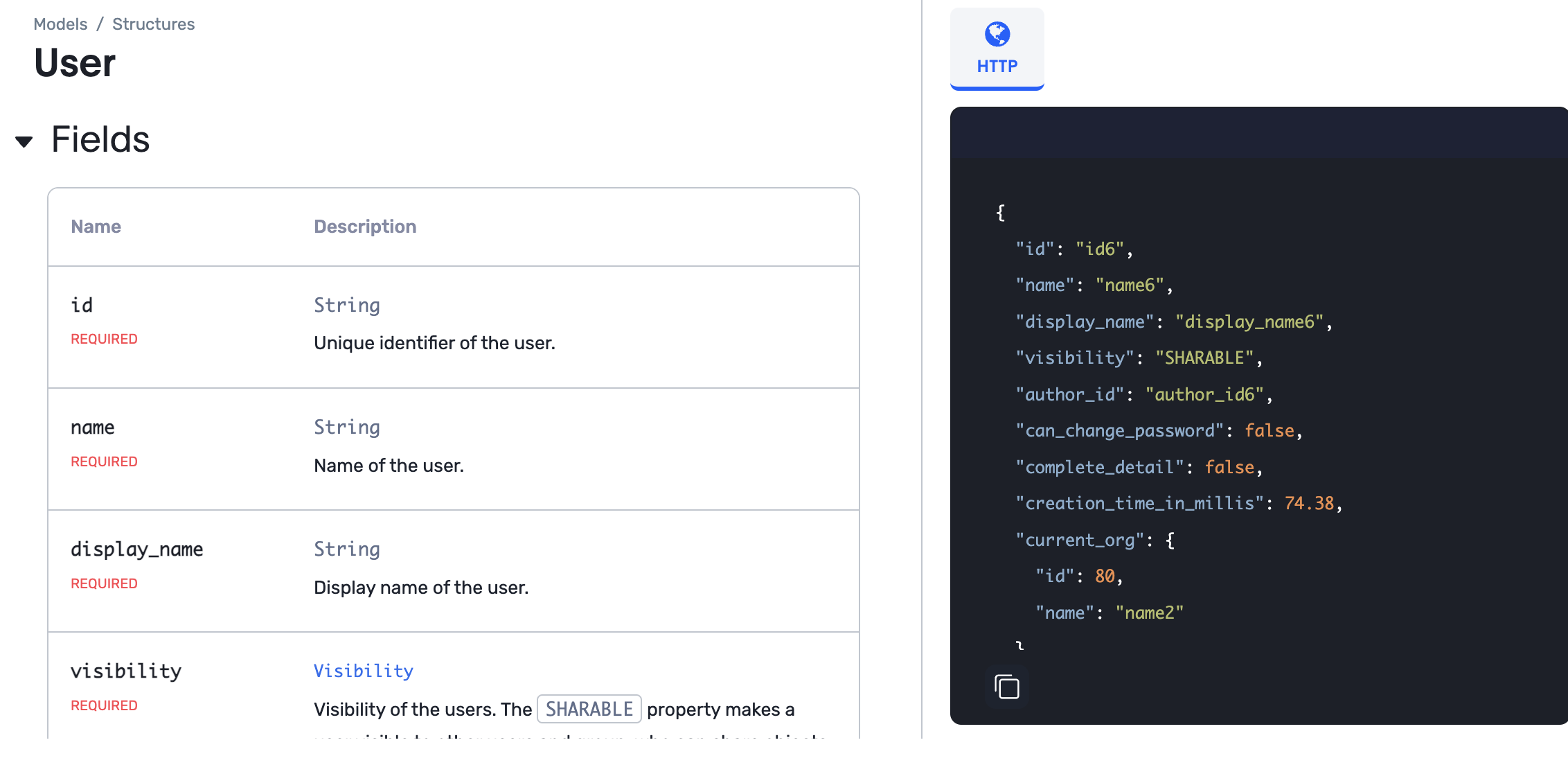
Note that the model and example will only be a singular form of the object that is returned.
If you look at the image above, the response is described as an Array<User>
, and so would be a JSON array containing any number of the User
model from the example.